pagehelper插件进行分页
创建项目
第一步(完成以下操作进行下一步):
" class="reference-link">第二步: 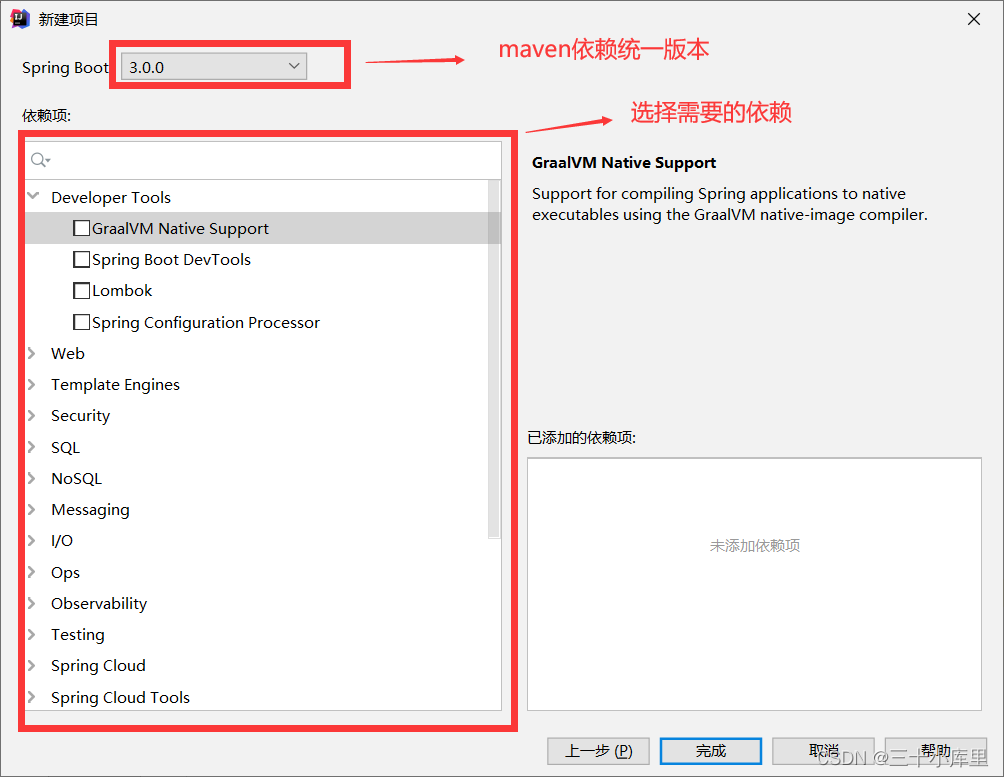
一、 原理概述
PageHelper是MyBatis的一个插件,内部实现了一个PageInterceptor拦截器。Mybatis会加载这个拦截器到拦截器链中。在我们使用过程中先使用PageHelper.startPage这样的语句在当前线程上下文中设置一个ThreadLocal变量,再利用PageInterceptor这个分页拦截器拦截,从ThreadLocal中拿到分页的信息,如果有分页信息拼装分页SQL(limit语句等)进行分页查询,最后再把ThreadLocal中的东西清除掉。
二、 springboot+pageHelper带条件分页
2.1 添加依赖
<dependency>
<groupId>com.github.pagehelper</groupId>
<artifactId>pagehelper-spring-boot-starter</artifactId>
<version>1.4.1</version>
</dependency>
2.2 pageHelper分页插件的yml配置
#pageHelper 分页插件的配置
pagehelper:
auto-dialect: true
reasonable: true
support-methods-arguments: true
params: count=countSql
2.3 建立实体类
package com.boot.springboot1223.pojo;
import com.baomidou.mybatisplus.annotation.IdType;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import lombok.Data;
import java.io.Serializable;
import java.util.Date;
/**
*
* @TableName action
*/
@Data
@TableName("action")
public class Action{
/**
* 操作ID
*/
@TableId(type = IdType.AUTO)
private Integer actionId;
/**
* 订单编号
*/
private String orderSn;
/**
* 操作人
*/
private Integer actionUser;
/**
* 订单状态
*/
private Integer orderStatus;
/**
* 支付状态
*/
private Integer payStatus;
/**
* 配送状态
*/
private Integer shippingStatus;
/**
* 操作记录
*/
private String actionNote;
/**
* 操作时间
*/
private String actionTime;
/**
* 状态描述
*/
private String statusDesc;
/**
* 下单时间
*/
@TableField(exist = false)
private String orderTime;
}
2.4 mapper层 (数据持久层)
package com.boot.springboot1223.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.boot.springboot1223.pojo.Action;
import org.apache.ibatis.annotations.Mapper;
import java.util.List;
@Mapper
public interface ActionMapper extends BaseMapper<Action> {
/**
* 分页加模糊查询
* @param action
* @return
*/
List<Action> findPage(Action action);
}
2.5 service层 (业务逻辑层)
package com.boot.springboot1223.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.boot.springboot1223.pojo.Action;
import com.github.pagehelper.PageInfo;
import java.util.List;
public interface ActionService extends IService<Action> {
PageInfo<Action> findPage(Action action,Integer pageIndex,Integer pageSize);
}
package com.boot.springboot1223.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.boot.springboot1223.mapper.ActionMapper;
import com.boot.springboot1223.pojo.Action;
import com.boot.springboot1223.service.ActionService;
import com.github.pagehelper.PageHelper;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
/**
* @Author
* @Date 2022/12/23
* @Description 类功能描述
*/
@Service
public class ActionServiceImpl extends ServiceImpl<ActionMapper, Action> implements ActionService {
@Autowired
private ActionMapper actionMapper;
@Override
public PageInfo<Action> findPage(Action action, Integer pageIndex, Integer pageSize) {
//调用分页插件的工具类 计算总页数
PageHelper.startPage(pageIndex,pageSize);
//获取所有数据
List<Action> page = actionMapper.findPage(action);
//获取所有的数据直接给pageInfo
PageInfo pageInfo=new PageInfo(page);
return pageInfo;
}
}
2.6 controller层
package com.boot.springboot1223.controller;
import com.boot.springboot1223.pojo.Action;
import com.boot.springboot1223.pojo.Order;
import com.boot.springboot1223.service.ActionService;
import com.boot.springboot1223.service.OrderService;
import com.github.pagehelper.PageInfo;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
/**
* @Author
* @Date 2022/12/23
* @Description 类功能描述
*/
@Controller
public class ActionController {
@Autowired
private ActionService actionService;
@RequestMapping("/findPage")
public String findPage(
Action action,
@RequestParam(value = "pageIndex",defaultValue = "1") Integer pageIndex,
@RequestParam(value = "pageSize",defaultValue = "1",required = false) Integer pageSize,
Model model
){
PageInfo<Action> page = actionService.findPage(action,pageIndex, pageSize);
model.addAttribute("path","findPage?pageIndex=");
model.addAttribute("page",page);
model.addAttribute("action",action);
return "list";
}
}
页面显示
还没有评论,来说两句吧...