Spring集成Redis
Spring-data-redis是spring大家族的一部分,提供了在srping应用中通过简单的配置访问redis服务,对reids底层开发包(Jedis, JRedis, and RJC)进行了高度封装,RedisTemplate提供了redis各种操作、异常处理及序列化,支持发布订阅,并对spring 3.1 cache进行了实现。
spring-data-redis针对jedis提供了如下功能:
- 连接池自动管理,提供了一个高度封装的“RedisTemplate”类
- 针对jedis客户端中大量api进行了归类封装,将同一类型操作封装为operation接口
- ValueOperations:简单K-V操作
- SetOperations:set类型数据操作
- ZSetOperations:zset类型数据操作
- HashOperations:针对map类型的数据操作
- ListOperations:针对list类型的数据操作
一、引入依赖
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.7.0</version>
</dependency>
<dependency>
<groupId>org.springframework.data</groupId>
<artifactId>spring-data-redis</artifactId>
<version>2.5.4</version>
</dependency>
如果存储的是Map<String,Object>需要导入jackson相关的包,存储的时候使用json序列化器存储。如果不导入jackson的包会报错。
<!-- springMVC支持json包-->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>2.10.0</version>
</dependency>
二、配置文件
(1) redis.properties
#redis集群
redis.host=192.168.147.128
redis.port=6379
#
redis.host2=192.168.147.128
redis.port2=6380
#
redis.host3=192.168.147.128
redis.port3=6381
#
redis.host4=192.168.147.128
redis.port4=6382
# 注意,如果没有password,此处不设置值,但这一项要保留
redis.password=
#最大空闲数,数据库连接的最大空闲时间。超过空闲时间,数据库连接将被标记为不可用,然后被释放。设为0表示无限制。
redis.maxIdle=300
#连接池的最大数据库连接数。设为0表示无限制
redis.maxActive=600
#最大建立连接等待时间。如果超过此时间将接到异常。设为-1表示无限制。
redis.maxWait=1000
#在borrow一个jedis实例时,是否提前进行alidate操作;如果为true,则得到的jedis实例均是可用的;
redis.testOnBorrow=true
(2)applicationContext-redis.xml
<!-- 连接池基本参数配置,类似数据库连接池 -->
<context:property-placeholder location="classpath:redis.properties"
ignore-unresolvable="true" />
<!-- redis连接池 -->
<bean id="poolConfig" class="redis.clients.jedis.JedisPoolConfig">
<property name="maxTotal" value="${redis.maxActive}" />
<property name="maxIdle" value="${redis.maxIdle}" />
<property name="testOnBorrow" value="${redis.testOnBorrow}" />
</bean>
<!-- redis集群 -->
<bean id="redisClusterConfiguration" class="org.springframework.data.redis.connection.RedisClusterConfiguration">
<property name="clusterNodes">
<set>
<bean class="org.springframework.data.redis.connection.RedisNode">
<constructor-arg name="host" value="${redis.host}"></constructor-arg>
<constructor-arg name="port" value="${redis.port}" />
</bean>
<bean class="org.springframework.data.redis.connection.RedisNode">
<constructor-arg name="host" value="${redis.host2}"></constructor-arg>
<constructor-arg name="port" value="${redis.port2}" />
</bean>
<bean class="org.springframework.data.redis.connection.RedisNode">
<constructor-arg name="host" value="${redis.host3}"></constructor-arg>
<constructor-arg name="port" value="${redis.port3}" />
</bean>
<bean class="org.springframework.data.redis.connection.RedisNode">
<constructor-arg name="host" value="${redis.host4}"></constructor-arg>
<constructor-arg name="port" value="${redis.port4}" />
</bean>
</set>
</property>
</bean>
<!-- 连接池配置,类似数据库连接池 -->
<bean id="jedisConnectionFactory"
class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
<constructor-arg name="clusterConfig" ref="redisClusterConfiguration"></constructor-arg>
<constructor-arg name="poolConfig" ref="poolConfig"></constructor-arg>
</bean>
<!--redis操作模版,使用该对象可以操作redis -->
<bean id="redisTemplate" class="org.springframework.data.redis.core.RedisTemplate" >
<property name="connectionFactory" ref="jedisConnectionFactory" />
<!--如果不配置Serializer,那么存储的时候缺省使用String,如果用User类型存储,那么会提示错误User can't cast to String!! -->
<property name="keySerializer" >
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<property name="valueSerializer" >
<bean class="org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer" />
</property>
<property name="hashKeySerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer"/>
</property>
<property name="hashValueSerializer">
<bean class="org.springframework.data.redis.serializer.GenericJackson2JsonRedisSerializer"/>
</property>
<!--开启事务 -->
<property name="enableTransactionSupport" value="true"></property>
</bean >
如果在多个spring配置文件中引入<context:property-placeholder .../>标签,最后需要加上ignore-unresolvable="true",否则会报错。
(3)测试
@Test
public void test() {
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext-redis.xml");
RedisTemplate redisTemplate = context.getBean("redisTemplate", RedisTemplate.class);
// stringRedisTemplate的操作
// String读写
redisTemplate.delete("k1");
redisTemplate.opsForValue().set("k1", "v1");
System.out.println(redisTemplate.opsForValue().get("k1"));
System.out.println("==============");
// List读写
redisTemplate.delete("l1");
redisTemplate.opsForList().rightPush("l1", "1");
redisTemplate.opsForList().rightPush("l1", "2");
redisTemplate.opsForList().leftPush("l1", "3");
List<String> listCache = redisTemplate.opsForList().range("l1", 0, -1);
for (String s : listCache) {
System.out.println(s);
}
System.out.println("==============");
// Set读写
redisTemplate.delete("s1");
redisTemplate.opsForSet().add("s1", "A");
redisTemplate.opsForSet().add("s1", "B");
redisTemplate.opsForSet().add("s1", "C");
Set<String> setCache = redisTemplate.opsForSet().members("s1");
for (String s : setCache) {
System.out.println(s);
}
System.out.println("===============");
// Hash读写
redisTemplate.delete("h1");
redisTemplate.opsForHash().put("h1", "address1", "北京");
redisTemplate.opsForHash().put("h1", "address2", "上海");
redisTemplate.opsForHash().put("h1", "address3", "河南");
Map<String, String> hashCache = redisTemplate.opsForHash().entries("h1");
for (Map.Entry entry : hashCache.entrySet()) {
System.out.println(entry.getKey() + "-" + entry.getValue());
}
System.out.println("===============");
}
执行结果
" class="reference-link">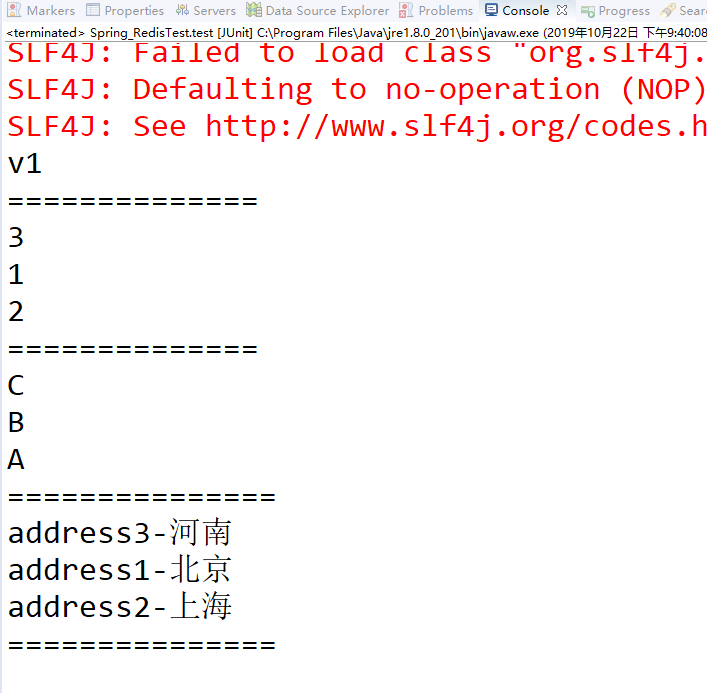
如果本文对您有很大的帮助,还请点赞关注一下。
还没有评论,来说两句吧...