python数字图像处理
1, subplot的使用
- matlab中的用法: subplot(m,n,p)或者subplot(m n p)
subplot是将多个图画到一个平面上的工具。其中,m和n代表在一个图像窗口中显示m行n列个图像,也就是整个figure中有n个图是排成一行的,一共m行,后面的p代表现在选定第p个图像区域,即在第p个区域作图。如果m=2就是表示2行图。p表示图所在的位置,p=1表示从左到右从上到下的第一个位置。
'''
注:ezplot(f,[-3,3]),表示画f函数的图形,取值区间在[-3,3]
代码如下:
'''
subplot(2,2,[1,2])
ezplot('sin',[-1,1])
grid minor
subplot(2,2,3)
ezplot('x',[-3,3])
subplot(2,2,4)
ezplot('x.^3',[-3,3])
grid
- matplotlib 中的subplot的用法: subplot(numRows, numCols, plotNum)
https://blog.csdn.net/haikuotiankong7/article/details/90551841
Matplotlib的子图subplot的使用https://www.jianshu.com/p/de223a79217a
matplotlib 中的subplot的用法https://www.cnblogs.com/caizhou520/p/11224526.html
import matplotlib.pyplot as plt
import numpy as np
def f(t):
return np.exp(-t) * np.cos(2 * np.pi * t)
if __name__ == '__main__' :
t1 = np.arange(0, 5, 0.1)
t2 = np.arange(0, 5, 0.02)
plt.figure(12)
plt.subplot(221)
plt.plot(t1, f(t1), 'bo', t2, f(t2), 'r--')
plt.subplot(222)
plt.plot(t2, np.cos(2 * np.pi * t2), 'r--')
plt.subplot(212)
plt.plot([1, 2, 3, 4], [1, 4, 9, 16])
plt.show()
2,颜色反转Grayscale conversion和cmap的取值
plt.imshow(data, cmap='Greens') #cmap是colormap的简称,用于指定渐变色,默认的值为viridis, 在matplotlib中,内置了一系列的渐变色.
"""
==================
Colormap reference:https://matplotlib.org/examples/color/colormaps_reference.html
==================
Reference for colormaps included with Matplotlib.
This reference example shows all colormaps included with Matplotlib. Note that
any colormap listed here can be reversed by appending "_r" (e.g., "pink_r").
These colormaps are divided into the following categories:
Sequential:
These colormaps are approximately monochromatic colormaps varying smoothly
between two color tones---usually from low saturation (e.g. white) to high
saturation (e.g. a bright blue). Sequential colormaps are ideal for
representing most scientific data since they show a clear progression from
low-to-high values.
Diverging:
These colormaps have a median value (usually light in color) and vary
smoothly to two different color tones at high and low values. Diverging
colormaps are ideal when your data has a median value that is significant
(e.g. 0, such that positive and negative values are represented by
different colors of the colormap).
Qualitative:
These colormaps vary rapidly in color. Qualitative colormaps are useful for
choosing a set of discrete colors. For example::
color_list = plt.cm.Set3(np.linspace(0, 1, 12))
gives a list of RGB colors that are good for plotting a series of lines on
a dark background.
Miscellaneous:
Colormaps that don't fit into the categories above.
"""
import numpy as np
import matplotlib.pyplot as plt
# Have colormaps separated into categories:
# http://matplotlib.org/examples/color/colormaps_reference.html
cmaps = [('Perceptually Uniform Sequential', [
'viridis', 'plasma', 'inferno', 'magma']),
('Sequential', [
'Greys', 'Purples', 'Blues', 'Greens', 'Oranges', 'Reds',
'YlOrBr', 'YlOrRd', 'OrRd', 'PuRd', 'RdPu', 'BuPu',
'GnBu', 'PuBu', 'YlGnBu', 'PuBuGn', 'BuGn', 'YlGn']),
('Sequential (2)', [
'binary', 'gist_yarg', 'gist_gray', 'gray', 'bone', 'pink',
'spring', 'summer', 'autumn', 'winter', 'cool', 'Wistia',
'hot', 'afmhot', 'gist_heat', 'copper']),
('Diverging', [
'PiYG', 'PRGn', 'BrBG', 'PuOr', 'RdGy', 'RdBu',
'RdYlBu', 'RdYlGn', 'Spectral', 'coolwarm', 'bwr', 'seismic']),
('Qualitative', [
'Pastel1', 'Pastel2', 'Paired', 'Accent',
'Dark2', 'Set1', 'Set2', 'Set3',
'tab10', 'tab20', 'tab20b', 'tab20c']),
('Miscellaneous', [
'flag', 'prism', 'ocean', 'gist_earth', 'terrain', 'gist_stern',
'gnuplot', 'gnuplot2', 'CMRmap', 'cubehelix', 'brg', 'hsv',
'gist_rainbow', 'rainbow', 'jet', 'nipy_spectral', 'gist_ncar'])]
nrows = max(len(cmap_list) for cmap_category, cmap_list in cmaps)
gradient = np.linspace(0, 1, 256)
gradient = np.vstack((gradient, gradient))
def plot_color_gradients(cmap_category, cmap_list, nrows):
fig, axes = plt.subplots(nrows=nrows)
fig.subplots_adjust(top=0.95, bottom=0.01, left=0.2, right=0.99)
axes[0].set_title(cmap_category + ' colormaps', fontsize=14)
for ax, name in zip(axes, cmap_list):
ax.imshow(gradient, aspect='auto', cmap=plt.get_cmap(name))
pos = list(ax.get_position().bounds)
x_text = pos[0] - 0.01
y_text = pos[1] + pos[3]/2.
fig.text(x_text, y_text, name, va='center', ha='right', fontsize=10)
# Turn off *all* ticks & spines, not just the ones with colormaps.
for ax in axes:
ax.set_axis_off()
for cmap_category, cmap_list in cmaps:
plot_color_gradients(cmap_category, cmap_list, nrows)
plt.show()
下面第一幅是用Greys, 第二幅是用Greys_r :
3,matplotlib.pyplot.axis()用法
axis()的用法https://www.cnblogs.com/10439-y/p/11788979.html
https://blog.csdn.net/muyimo/article/details/79697905
matplotlib.pyplot.axis() https://blog.csdn.net/The_Time_Runner/article/details/89888206
4,matplotlib中的plt.figure()、plt.subplot()、plt.subplots()、add_subplots以及add_axes的使用
注意:subplot和subplots的区别
https://blog.csdn.net/C_chuxin/article/details/83994457
python3_matplotlib_figure()函数解析https://blog.csdn.net/admin_maxin/article/details/80667671
python中关于matplotlib库的figure,add_subplot,subplot,subplots函数https://blog.csdn.net/weixin_44922452/article/details/90716289
5,python3,matplotlib绘图,title、xlabel、ylabel、图例等出现中文乱码
https://blog.csdn.net/weixin_43186036/article/details/82881748
在Windows系统找到中文字体添加进去就可以,只有部分字体能够右键查看他们的属性\Windows\Fonts
'''
常用字体对应路径
宋体常规 C:\Windows\Fonts\simsun.ttc
新宋体常规 C:\Windows\Fonts\simsun.ttc
黑体常规 C:\Windows\Fonts\simhei.ttf
楷体常规 C:\Windows\Fonts\simkai.ttf
仿宋常规 C:\Windows\Fonts\simfang.ttf
隶体常规 C:\Windows\Fonts\SIMLI.TTF
华文新魏 C:\Windows\Fonts\STXINWEI.TTF
'''
'''
%matplotlib inline 可以在Ipython编译器里直接使用,功能是可以内嵌绘图,并且可以省略掉plt.show()这一步。
但在spyder用会报错. 经过我的的验证该句在spyder下的Ipython也没有任何作用,因为在spyder下的Ipython会自动输出图像
'''
%matplotlib inline
import matplotlib # 注意这个也要import一次
import matplotlib.pyplot as plt
#from matplotlib.font_manager import FontProperties
#font_set = FontProperties(fname=r"c:/windows/fonts/simsun.ttc", size=15)
'''
路径中的双引号和单引号都可以,斜杠和反斜杠也都可以
另外说明:因python3默认使用中unicode编码, 所以在写代码时不再需要写 plt.xlabel(u'横'),而是直接写plt.xlabel('横')
'''
#myfont = matplotlib.font_manager.FontProperties(fname=r"C:\Windows\Fonts\STXINWEI.TTF") # fname指定字体文件 选简体显示中文
myfont = matplotlib.font_manager.FontProperties(fname=r'C:\Windows\Fonts\simkai.ttf', size=15)
plt.plot((1,2,3),(4,3,-1))
plt.xlabel('横', fontproperties=myfont) # 这一段
plt.ylabel('纵', fontproperties=myfont) # 这一段
#plt.show() # 有了%matplotlib inline 就可以省掉plt.show()了
'''
也可以不使用matplotlib.font_manager.FontProperties语句导入字体,直接使用fontproperties='SimHei',matplotlib可以自己找到部分字体,但找不全
'''
plt.plot((1,2,3),(4,3,-1))
plt.xlabel(u'横坐标', fontproperties='STXINWEI') #新魏字体
plt.ylabel(u'纵坐标', fontproperties='SimHei') # 黑体
6,matplotlib基础绘图命令之imshow
https://cloud.tencent.com/developer/article/1677118
7,用箭头和文字来标记重要的点,对图标的坐标轴进行调整
https://cloud.tencent.com/developer/article/1688511
https://cloud.tencent.com/developer/article/1688564
8,python画图入门汇总教程-先看
Python3快速入门(十六)——Matplotlib绘图https://blog.51cto.com/9291927/2435621
python画图入门https://zhuanlan.zhihu.com/c_1239148130719158272
9,python:图片的float类型和uint8类型
https://blog.csdn.net/weixin_40522801/article/details/107901533
python中不同函数读取图片格式的区别https://blog.csdn.net/u013044310/article/details/80739623
在python图像处理过程中,遇到的RGB图像的值是处于0-255之间的,为了更好的处理图像,通常python提供的读取图片函数默认都会将图像值转变到0-1之间.
float,float32,float64这三个数据类型的范围都是 [0.0, 1.0] or [-1.0, 1.0]
//scikit-image.org/docs/stable/api/skimage.util.html
10,scipy.misc.bytescale的替代方案
scipy.misc.bytescale的替代函数代码https://download.csdn.net/download/king52113141314/12810274
skimage.util.img_as_ubyte
is a replacement forscipy.misc.bytescale
the
scipy.misc.bytescale
doc states the following:Byte scaling means converting the input image to uint8 dtype and scaling the range to (low, high) (default 0-255). If the input image already has dtype uint8, no scaling is done.
the
skimage.util.img_as_ubyte
doc states the following:Convert an image to 8-bit unsigned integer format. Negative input values will be clipped. Positive values are scaled between 0 and 255.
https://stackoverflow.com/questions/58811889/what-replaces-scipy-misc-bytescale
https://stackoverflow.com/questions/53705804/alternatives-to-scipys-misc-bytescale-method
11,matplotlib.pyplot.``imread函数返回值
https://matplotlib.org/3.1.1/api/_as_gen/matplotlib.pyplot.imread.html
Returns: | imagedata : The image data. The returned array has shape
|
---|
12,用python将图像转换为三维数组
RGB images are usually stored as 3 dimensional arrays of 8-bit unsigned integers. The shape of the array is:
height x width x 3. 这和分辨率(1920×1080)恰相反
用python将图像转换为三维数组之后,每一维,每个元素值都代表着什么?https://blog.csdn.net/gaifuxi9518/article/details/88364167
13, Creating Image with numpy
在线拾色器-输入RGB值输出颜色https://www.w3cschool.cn/tools/index?name=cpicker
https://www.pythoninformer.com/python-libraries/numpy/numpy-and-images/
14,numpy之histogram,plt.bar()与plt.barh条形图
https://blog.csdn.net/yangwangnndd/article/details/89489946
plt.bar()与plt.barh条形图https://www.cnblogs.com/zyg123/p/10504637.html
numpy.``histogram
(a, bins=10, range=None, normed=False, weights=None, density=None)[source]
Returns: hist : array
The values of the histogram. See density and weights for a description of the possible semantics.
bin_edges : array of dtype float
Return the bin edges
(length(hist)+1)
a是待统计数据的数组;
bins指定统计的区间个数;
range是一个长度为2的元组,表示统计范围的最小值和最大值,默认值None,表示范围由数据的范围决定
weights为数组的每个元素指定了权值,histogram()会对区间中数组所对应的权值进行求和
density为True时,返回每个区间的概率密度;为False,返回每个区间中元素的个数
import numpy as np
import matplotlib.pyplot as plt
a = np.random.rand(100)
np.histogram(a,bins=5,range=(0,1))
dct_hist, dct_bin_edges = np.histogram(a,bins=5,range=(0,1))
img_hist, img_bin_edges = np.histogram(a,bins=[0,0.2,0.5,0.8,1])
f, (plt1, plt2) = plt.subplots(1, 2, figsize=(15, 5))
plt1.set_title('Frequency histogram')
plt1.bar(dct_bin_edges[:-1], dct_hist, width = 0.1)#注意dct_bin_edges的数据比dct_hist数据多一个,因此要去除一个数据才能画图
plt2.set_title('Spatial histogram')
plt2.bar(img_bin_edges[1:], img_hist, width = 0.1)
#输出
In [75]: np.histogram(a,bins=5,range=(0,1))
Out[75]:
(array([18, 19, 13, 24, 26], dtype=int64),#注意bin_edges的数据比hist数据多一个,即the bin edges (length(hist)+1)
array([0. , 0.2, 0.4, 0.6, 0.8, 1. ])) #18表示a中有18个数落在[0,0.2]区间,19个数落在[0.2,0.4]区间
In [76]: np.histogram(a,bins=[0,0.2,0.5,0.8,1])
Out[76]: (array([18, 26, 30, 26], dtype=int64), array([0. , 0.2, 0.5, 0.8, 1. ]))
" class="reference-link">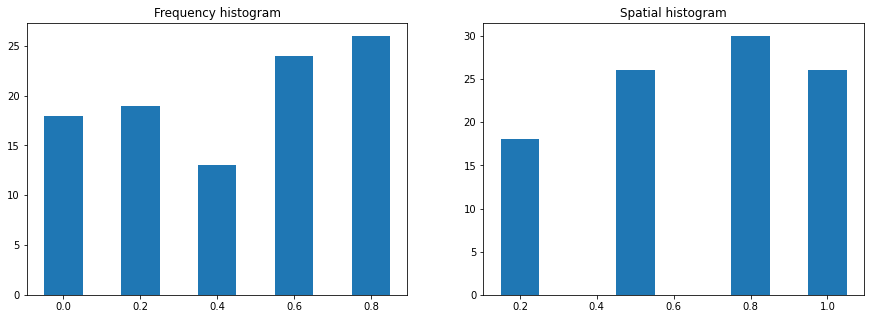
15,Python中[ : n]、[m : ]、[-1]、[:-1]、[::-1]、[2::-1]和[1:]的含义
https://blog.csdn.net/SpringRolls/article/details/95220169
Python中双冒号的作用[::]
Python sequence slice addresses can be written as a[start
step] and any of start, stop or end can be dropped.
Python序列切片地址可以写为[开始:结束:步长],其中的开始和结束可以省略
#
#
#
#
#
#
#
#
#
#
参考:
python数字图像处理 https://www.cnblogs.com/denny402/p/5121501.html
python skimage图像处理(一)https://www.jianshu.com/p/f2e88197e81d
用python简单处理图片(1):打开\显示\保存图像 https://www.cnblogs.com/denny402/p/5096001.html
「Matplotlib绘图详解」https://zhuanlan.zhihu.com/p/43877804
Matplotlib 教程https://www.runoob.com/w3cnote/matplotlib-tutorial.html
Matplotlib official tutorial https://matplotlib.org/tutorials/colors/colormaps.html
python之matplotlib绘图基础 https://www.cnblogs.com/liutongqing/p/6985805.html
还没有评论,来说两句吧...